[FastAPI] Project2 - Move Fast with FastAPI
<span style="color:grey">이 포스트는 유데미 강의 FastAPI 강의를 듣고 정리한 글입니다.'</span>
## Project2 개요
이번 시간에는 지난번에 이어 book project2 를 진행할 계획이다.
GET, POST, PUT, DELETE 리퀘스트 메소드는 계속 사용하되
프로젝트2에서는 데이터 유효성 검사(Data Validation) , 예외 처리(Exception Handling), 상태 코드 (Status Codes), 스웨거 Configuration, 파이썬 리퀘스트 객체에 대해 추가적으로 학습할 것이다.
프로젝트1에서 만든 book 이 프로젝트2에서는 파이썬 객체가 될 것이다. 그렇가 하려면 Book 이라는 새로운 **"클래스"** 를 생성해야 한다.
우리는 이 프로젝트를 통해 이러한 book들을 이용하게 될 것이다.
book 오브젝트를 만들때는 아래 코드를 참고하면 알 수 있듯이, 생성자를 통해 생성할 수 있다.
```
class Book:
id: int
title: str
author: str
description: str
rating: int
def __init__(self, id, title, author, description, rating):
self.id = id
self.title = title
self.author = author
self.description = description
self.rating = rating
```
### Pydantic v1 vs Pydantic v2 이슈
> - FastAPI is now compatible with both Pydantic v1 and Pydantic v2.
Based on how new the version of FastAPI you are using, there could be small method name changes.
The three biggest are:
> .dict() function is now renamed to .model_dump()
schema_extra function within a Config class is now renamed to json_schema_extra
Optional variables need a =None example: id: Optional[int] = None
=> 정리하면 FastAPI 가 현재 Pydantic v1 과 v2 둘다 호환가능해서 FastAPI 버전에 따라 메소드 이름이 바뀔 수 있다는 것.
예를 들어 .dic() 함수는 .model_dump() 로 바뀌었고
schema_extra function 은 json_schema_extra 로
바뀌었다.
## Project2 SetUP
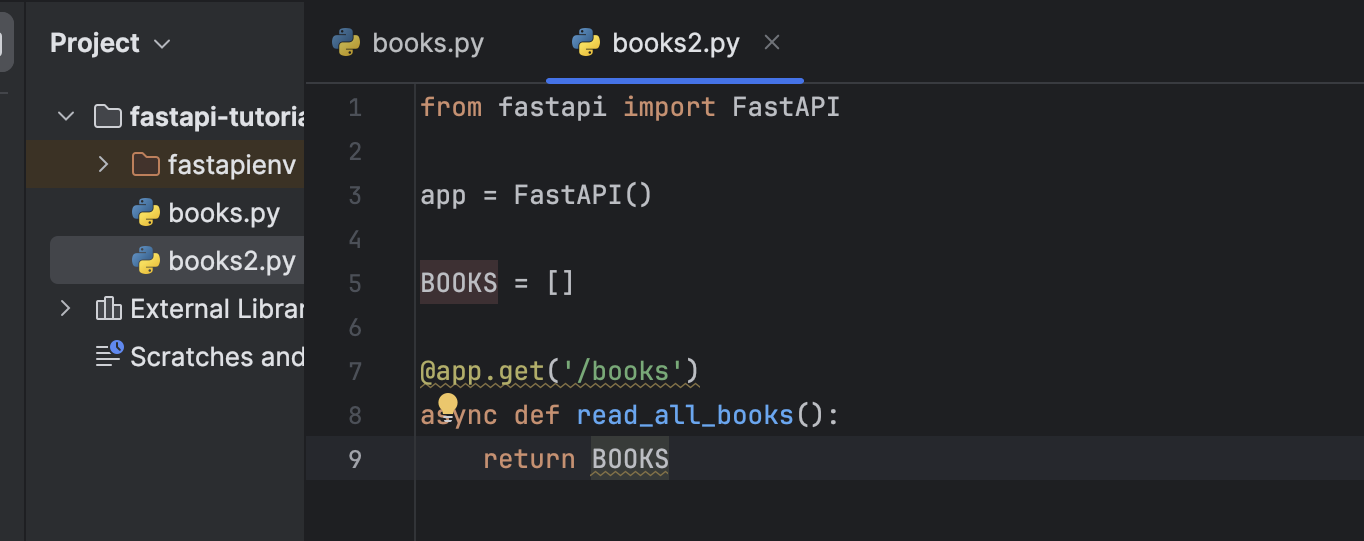
프로젝트 2 는 새로운 파이썬 파일로 작성할 것이기 때문에 books2.py 를 새롭게 생성한 후, 초기 설정은 그대로 진행한다.
```
from fastapi import FastAPI
# fastapi 에서 FastAPI 임포트하기
app = FastAPI()
#FastAPI 객체 생성 후 app 에 담기
@app.get('/books')
async def read_all_books():
return BOOKS
# books 경로 진입시 모든 책을 GET 하는 함수를 정의한다.
```
다음은 books2.py 에서는 BOOK 클래스를 통해 객체를 생성할 것이기 때문에 app 밑에 BOOK 클래스를 정의한다.
```
class Book:
id: int
title: str
author: str
description: str
rating: int
def __init__(self, id, title, author, description, rating):
self.id = id
self.title = title
self.author = author
self.description = description
self.rating = rating
```
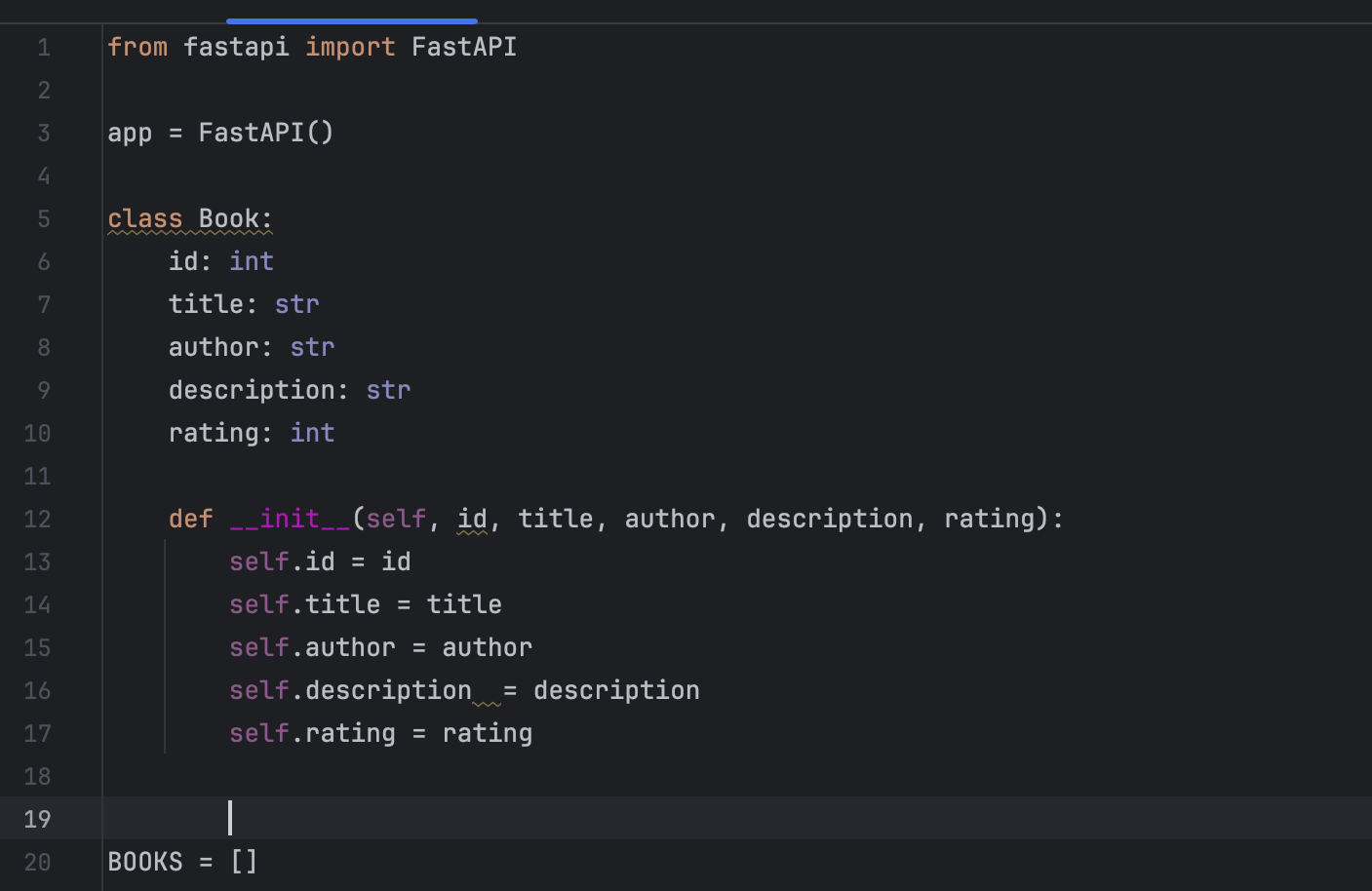
BOOK 오브젝트들은 강의 내용에 나온대로 작성하였다.
```
BOOKS = [
Book(1, 'Computer Science Pro', 'codingwithroby', 'A very nice book', 5),
Book(2, 'Be Fast with FastAPI', 'codingwithroby', 'A very great book', 5),
Book(3, 'Master Endpoints', 'codingwithroby', 'A awesome book', 5),
Book(4, 'HP1', 'Author 1', 'Book Description', 2),
Book(5, 'HP2', 'Author 2', 'Book Description', 3),
Book(6, 'HP3', 'Author 3', 'Book Description', 1),
]
```
## Project2 SetUP